Beautiful Plants For Your Interior
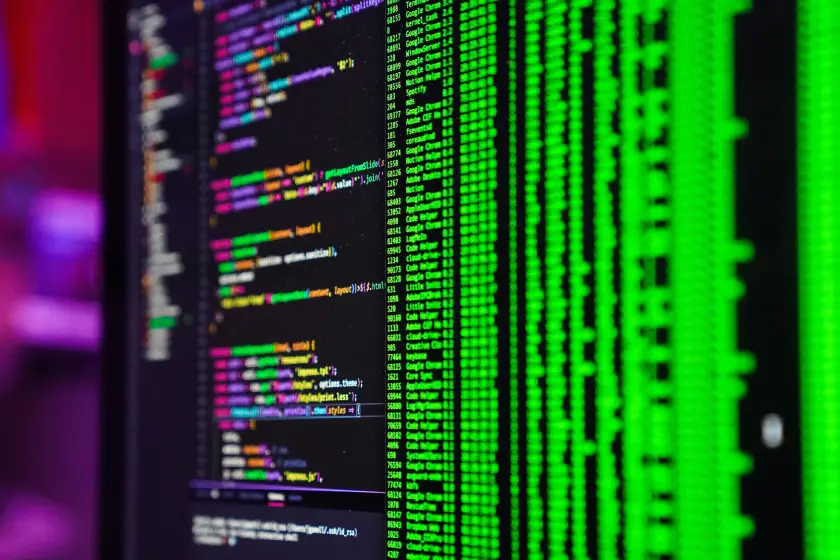
Learn how to build a Next.js app from scratch with this detailed guide. Perfect for beginners and experienced developers, it covers everything from setup to deployment, ensuring a high-performance, SEO-friendly app.
How to build a Next.js App involves several steps, from setting up the project to deploying it. Here’s a step-by-step guide to help you get started:
Step 1: How to Build a Next.js App
1.1. Install Node.js if you haven’t already. You can download it from nodejs.org.
1.2. Create a new Next.js project:
npx create-next-app@latest my-blog
cd my-blog
1.3. Start the development server:
npm run dev
Step 2: Create a Blog Structure
1. Create a pages
directory: Inside the pages
directory, you can create subdirectories for your blog posts.
pages/
├── index.js
└── posts/
├── first-post.js
└── second-post.js
2. Create your blog posts: In posts/first-post.js
, add the following:
const FirstPost = () => {
return (
<div>
<h1>First Post</h1>
<p>This is my first blog post!</p>
</div>
);
};
export default FirstPost;
Repeat for second-post.js
.
Step 3: Dynamic Routing
Instead of creating separate files for each blog post, use dynamic routing.
1. Create a [slug].js
file: In pages/posts/
, create a file named [slug].js
.
2. Implement dynamic routing:
import { useRouter } from 'next/router';
const posts = {
first: { title: "First Post", content: "This is my first blog post!" },
second: { title: "Second Post", content: "This is my second blog post!" }
};
const Post = () => {
const router = useRouter();
const { slug } = router.query;
const post = posts[slug];
return (
<div>
<h1>{post.title}</h1>
<p>{post.content}</p>
</div>
);
};
export default Post;
Step 4: Create a Home Page
1. Edit index.js
to list your blog posts:
import Link from 'next/link';
const Home = () => {
return (
<div>
<h1>My Blog</h1>
<ul>
<li><Link href="/posts/first">First Post</Link></li>
<li><Link href="/posts/second">Second Post</Link></li>
</ul>
</div>
);
};
export default Home;
Step 5: Styling
1. Add Tailwind CSS for styling: Follow the official Tailwind CSS installation guide to integrate it with your Next.js project.
2. Style your blog using Tailwind classes: Update your components with Tailwind classes for better styling.
Step 6: Fetching Data (Optional)
If you want to fetch blog posts from an external source or a CMS, you can use getStaticProps
or getServerSideProps
.
Example for static generation in [slug].js
:
export async function getStaticPaths() {
return {
paths: [
{ params: { slug: 'first' } },
{ params: { slug: 'second' } },
],
fallback: false,
};
}
export async function getStaticProps({ params }) {
const post = posts[params.slug]; // Fetch post data
return {
props: {
post,
},
};
}
Step 7: Deploy Your Blog
1. Deploy to Vercel:
- Push your code to a GitHub repository.
- Go to Vercel, create an account, and import your GitHub repo.
- Follow the prompts to deploy your Next.js blog.
Conclusion
Congratulations! You now have a simple Next.js blog. You can expand on this by adding features like comments, tags, or a CMS for easier content management. Let me know if you need help with any specific parts!