Beautiful Plants For Your Interior
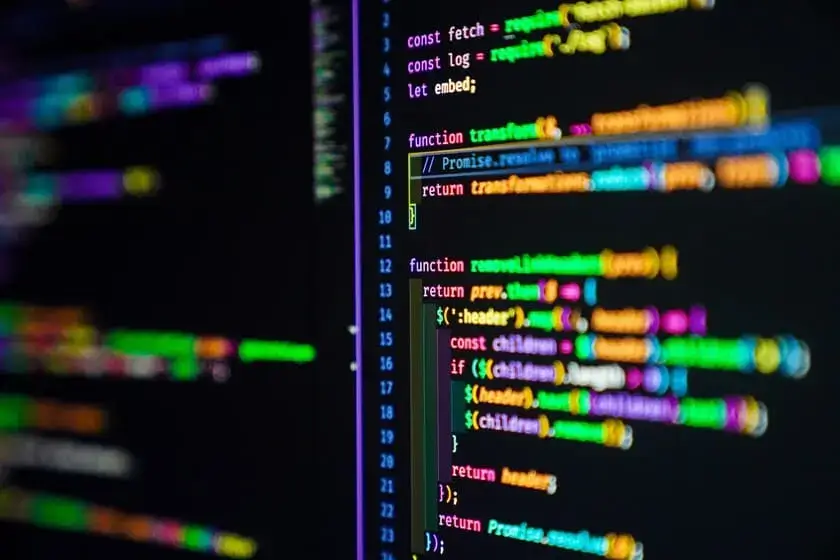
Three.js is a powerful JavaScript library for creating 3D content for web browsers using WebGL. Whether you’re building an interactive 3D model viewer or a full-fledged 3D game, Three.js simplifies the process. In this guide, we’ll cover the step-by-step process of installing three.js with npm and provide a simple example to get you started.
Step 1: Setting Up the Project
Before we install Three.js, you’ll need to create a new project folder for your 3D web application.
1. Create a directory: Open a terminal and create a folder for your project:
mkdir threejs-app
cd threejs-app
2. Initialize npm: Inside your project folder, run the following command to create a package.json
file, which will manage your project’s dependencies:
npm init -y
Step 2: Install three.js with npm
Once your project is set up, you can install three.js with npm.
1. Install Three.js: Run the following command in your terminal:
npm install three
This will download and install the Three.js library and list it as a dependency in your package.json
file.
Step 3: Set Up a Basic HTML File
Create an index.html
file to serve as the entry point for your 3D application. This file will include your JavaScript code that uses Three.js to render a simple 3D scene.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Three.js Example</title>
<style>
body { margin: 0; }
canvas { display: block; }
</style>
</head>
<body>
<script type="module" src="main.js"></script>
</body>
</html>
This file sets up the basic structure of an HTML document and links to a JavaScript file (main.js
) where we’ll write our Three.js code.
Step 4: Create a Basic Three.js Scene
Now, let’s write some JavaScript to create a 3D scene. In the same project folder, create a main.js
file:
// Import Three.js
import * as THREE from 'three';
// Set up the scene
const scene = new THREE.Scene();
// Create a camera
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
// Create a renderer and attach it to the document
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// Create a simple cube geometry
const geometry = new THREE.BoxGeometry();
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const cube = new THREE.Mesh(geometry, material);
// Add the cube to the scene
scene.add(cube);
// Position the camera
camera.position.z = 5;
// Animation loop
function animate() {
requestAnimationFrame(animate);
// Rotate the cube for some basic animation
cube.rotation.x += 0.01;
cube.rotation.y += 0.01;
// Render the scene
renderer.render(scene, camera);
}
// Start the animation loop
animate();
In this code:
- We import the necessary parts of the Three.js library.
- We create a scene, camera, and renderer.
- We create a simple cube using
BoxGeometry
and a greenMeshBasicMaterial
. - The cube is added to the scene, and the camera is positioned.
- Finally, we set up an animation loop that rotates the cube and re-renders the scene on each frame.
Step 5: Running the Project
If you open the index.html
file directly in the browser, you’ll notice the import
statement won’t work due to module restrictions in modern browsers. To fix this, we need to run the project on a local server.
1. Install a local development server: You can use http-server
, which is an easy-to-use static file server.
Install http-server
globally:
npm install -g http-server
2. Start the server: Once installed, you can run the server in your project directory:
http-server
3. Access your project: Open your browser and navigate to http://localhost:8080
. You should see a green, rotating cube on the screen!
Conclusion
With this setup, you’ve successfully installed three.js with npm and rendered a basic 3D cube in a web browser. Three.js offers countless possibilities for 3D graphics on the web, and this example is just the beginning. From here, you can explore more complex geometries, materials, lighting, and animations.
Feel free to experiment and build your own 3D web projects!